Run-Time Type Checking in TypeScript with io-ts
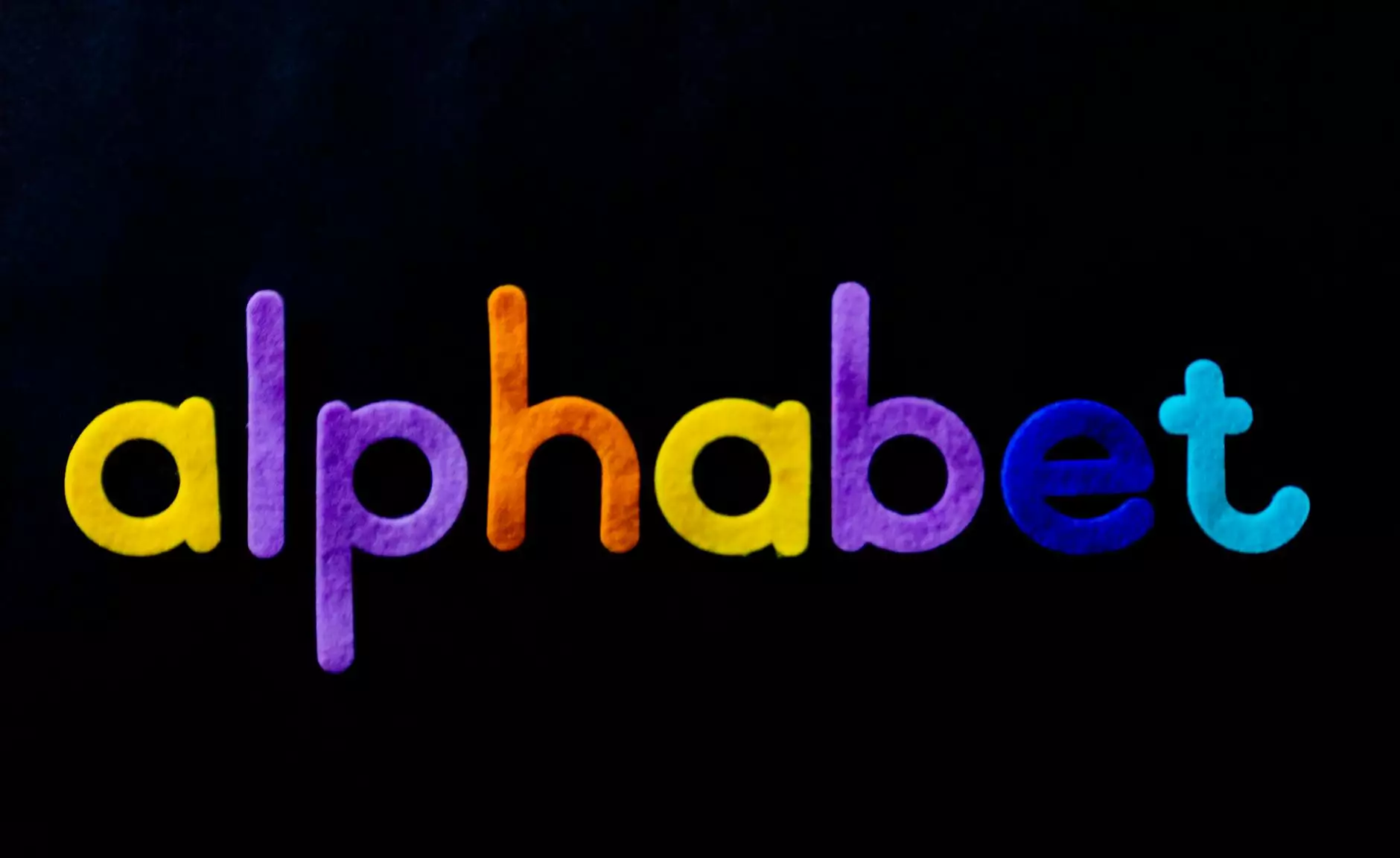
Introduction
Welcome to Newark SEO Experts, a leading provider of digital marketing services in the category of Business and Consumer Services. In this article, we will explore the concept of run-time type checking in TypeScript using the powerful library io-ts.
Understanding TypeScript and io-ts
TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. It provides optional static type checking, improving the reliability and maintainability of JavaScript projects.
io-ts is a runtime type system for TypeScript and JavaScript with a focus on providing a fast, convenient, and reliable way of defining complex data validations. It allows developers to define and enforce runtime type checks, ensuring data integrity and minimizing runtime errors.
Why Run-Time Type Checking?
Run-time type checking offers several benefits in software development:
- Enhanced Code Robustness: By verifying types at runtime, developers can catch potential errors early and prevent unexpected runtime exceptions.
- Improved Code Maintainability: With clearly defined types, it becomes easier to understand and refactor code, saving time and effort in future development cycles.
- Reduced Debugging Efforts: By eliminating common type-related issues, developers can focus more on the logic of their code rather than troubleshooting type mismatches.
Exploring io-ts Features
io-ts provides a rich set of features to define and validate complex data structures:
- Type Definitions: io-ts offers a wide range of built-in and customizable type definitions, such as primitive types, literals, arrays, objects, and more.
- Composition and Modularity: Types can be composed together to form more complex structures, enabling easy reuse and separation of concerns.
- Type Guards: io-ts allows the creation of type guards, which can be used to narrow down the types of values during runtime.
- Error Reporting: When a validation fails, io-ts provides detailed error messages, making it easier to identify and rectify the cause.
Example: Validating User Data
Let's consider a scenario where we need to validate user data before saving it to a database. With io-ts, we can define a type schema for the user data and perform runtime validations with ease.
Here's an example:
import * as t from 'io-ts'; const User = t.type({ id: t.number, name: t.string, email: t.string, age: t.union([t.number, t.null]), }); function saveUser(userData) { const validationResult = User.decode(userData); if (validationResult.isRight()) { // Valid user data // Save to the database } else { // Invalid user data console.error('Validation errors:', validationResult.left()); } }In the above code snippet, we define a type schema for a user with properties such as id, name, email, and age. By using the t.type and other io-ts combinators, we construct a robust type validation.
When the saveUser function receives user data, it attempts to decode and validate it against the defined User type schema. If the validation succeeds, the data is considered valid, and we can proceed to save it to the database. Otherwise, the function logs the validation errors to the console.
Conclusion
Run-time type checking with io-ts provides a powerful mechanism to ensure data integrity and prevent runtime errors in TypeScript and JavaScript projects. By leveraging the features of io-ts, developers can create robust and maintainable codebases, leading to enhanced software reliability and developer productivity.
Partner with Newark SEO Experts for all your digital marketing needs and stay ahead of the competition. Contact us today to learn more about our comprehensive services.
© 2020 Newark SEO Experts. All rights reserved.